Gradient TableView Cells
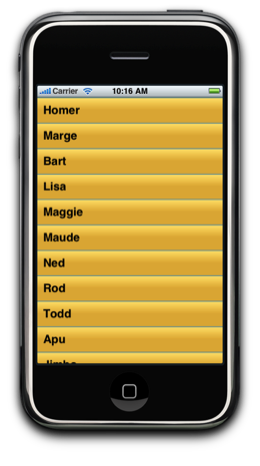
To the left is a screen shot of the finished application. At the end of the tutorial is the code to make the magic happen. You may use the code as you see fit in any application. If you intend distributing the source code please leave in the copyright notice.
Start by creating a new project in xcode. Select “View based application” and save the name as TableViewGradients.
Four Class files will have been created for you, and two resource files.
Add the UITableViewDelegate and UITableViewDataSource to TableViewGradientsViewController as shown below.
Now double click TableViewGradientsViewController.xib and open Interface Builder.
From the library drag and drop a Table View onto the view.
The next step is to assign the data source and data delegate for the table view. The data source defines where to get the data from to update the table. The delegate defines who is responsible for updating the display of the table.
To do this, right click on the table view and a popup menu will be displayed. Click on the circle on the far right for the dataSource and drag and drop onto the File's Owner. Do the same for the delegate.
Now save your changes in Interface Builder.
Time for some dummy data.
In TableViewGradientsViewController.h create an array to hold the data for the table.
From the library drag and drop a Table View onto the view.
The next step is to assign the data source and data delegate for the table view. The data source defines where to get the data from to update the table. The delegate defines who is responsible for updating the display of the table.
To do this, right click on the table view and a popup menu will be displayed. Click on the circle on the far right for the dataSource and drag and drop onto the File's Owner. Do the same for the delegate.
Now save your changes in Interface Builder.
Time for some dummy data.
In TableViewGradientsViewController.h create an array to hold the data for the table.
In TableViewGradientsViewController.m lets setup the data. We want to initialize the data before the view is displayed so un comment viewDidLoad and create the data for the array in this method. Here is some sample data.
The next step is to create the data source methods, there are only two methods that are required.
tableView:tableView:numberOfRowsInSection:section is pretty straight forward and simply returns the number of rows in the dataArray.
tableView:tableView:cellForRowAtIndexPath:indexPath is the method that ties the data to the cell and returns the cell for display. This is where we will add extra code to give it the "bling!".
tableView:tableView:cellForRowAtIndexPath:indexPath is the method that ties the data to the cell and returns the cell for display. This is where we will add extra code to give it the "bling!".
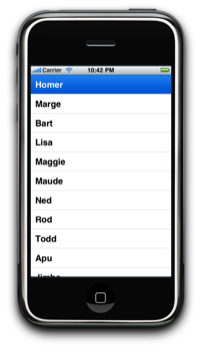
Before we do that build the app to make sure you have the current steps.
Your app should look like the image on the left. Now its time to add the bling!
You could simply add a flat color to the background of your tableview cells, but if you want some type of gradient you will need to create an image that can be placed in the cell background view.
If your table cells are all one size a background image is a viable option. But if your tableviewcells are of different heights then this will not work well as the image will have to be stretched or compressed to fit. With a small amount of effort we can use some methods from Core Animation to give a gradient to a cell of any size. We will just be using the CAGradientLayer class from Core Animation.
To use Core Animation we need to include the header file and add the framework to our project.
At the top of TableViewGradientsViewController.h add the line shown below.
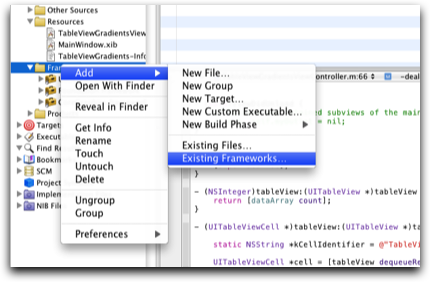
Now add the QuartzCore framework.
We now need to modify the method cellForRowAtIndexPath to use the Core Animation capabilities. Replace your method cellForRowAtIndexPath with the code shown below.
What are these extra lines of code doing?
First we create a frame that is the size of the cell. Here we are simply hard coding the height to be 44 pixels high.
We then set the cell frame to be the size of the frame we created and we create a new UIView of the same size.
In the Core Animation Framework there is an object type called CAGradientLayer which handles all the hard work of making the actual gradient color.
We create gradientLayer and set its size the same frame size as the UIView.
The next step is to place the gradientLayer in the cell. We are going to put the gradient layer in the middle of the cell.
The startPoint and the endPoint tell the gradientLayer where the color gradient is going to start and stop. We want the color to change vertically, we could have quite easily had the color change horizontally. The startPoint and endPoint are specified on the UNIT scale meaning 0,0 is the top left corner and 1,1 is the bottom right corner for our UIView.
The next step is to specify the colors in an array. The CAGradientLayer wants the colors in the CGColor format but as we are used to the UIColor methods we specify the colors and the alpha channel (transparency) and use a help macro to get the correct value.
You can have as many colors specified here as you want you could choose to go from red, to blue to orange to green. CAGradientLayer allows you total control over how the gradient is drawn. You may want the red section to be small followed by a large blue section. To specify how big each section is you give the starting position for the color. These values are given on the UNIT scale too.
You can see in the example that I specified the same color twice. So the color changes from 0xE5B422FF to 0xE5B422FF. That is because I wanted a solid color for the bottom half of the cell.
Add the macro below to the top of the file TableViewGradientsViewController.m The macro allows us to easily use a single hex values for the Red, Green, Blue and Alpha channels.
First we create a frame that is the size of the cell. Here we are simply hard coding the height to be 44 pixels high.
We then set the cell frame to be the size of the frame we created and we create a new UIView of the same size.
In the Core Animation Framework there is an object type called CAGradientLayer which handles all the hard work of making the actual gradient color.
We create gradientLayer and set its size the same frame size as the UIView.
The next step is to place the gradientLayer in the cell. We are going to put the gradient layer in the middle of the cell.
The startPoint and the endPoint tell the gradientLayer where the color gradient is going to start and stop. We want the color to change vertically, we could have quite easily had the color change horizontally. The startPoint and endPoint are specified on the UNIT scale meaning 0,0 is the top left corner and 1,1 is the bottom right corner for our UIView.
The next step is to specify the colors in an array. The CAGradientLayer wants the colors in the CGColor format but as we are used to the UIColor methods we specify the colors and the alpha channel (transparency) and use a help macro to get the correct value.
You can have as many colors specified here as you want you could choose to go from red, to blue to orange to green. CAGradientLayer allows you total control over how the gradient is drawn. You may want the red section to be small followed by a large blue section. To specify how big each section is you give the starting position for the color. These values are given on the UNIT scale too.
You can see in the example that I specified the same color twice. So the color changes from 0xE5B422FF to 0xE5B422FF. That is because I wanted a solid color for the bottom half of the cell.
Add the macro below to the top of the file TableViewGradientsViewController.m The macro allows us to easily use a single hex values for the Red, Green, Blue and Alpha channels.
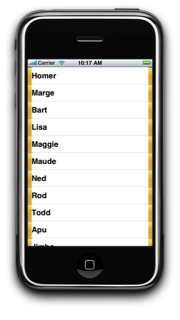
To be honest I am not 100% sure why the results look like this, but I do know how to fix it.
Open the file TableViewTricksViewController.xib in Interface Builder. Select the table view and then open the attributes inspector and change the separator to none and the background color an opacity of zero. Those two settings are shown in the image on the right.
Save your changes and rebuild the app, you should now have an app that looks like the first screen shot.
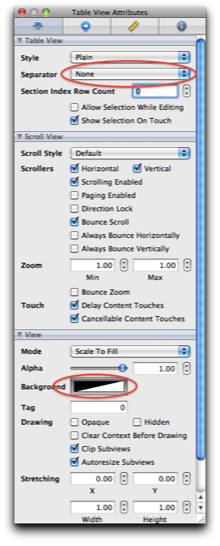